Higher order functions
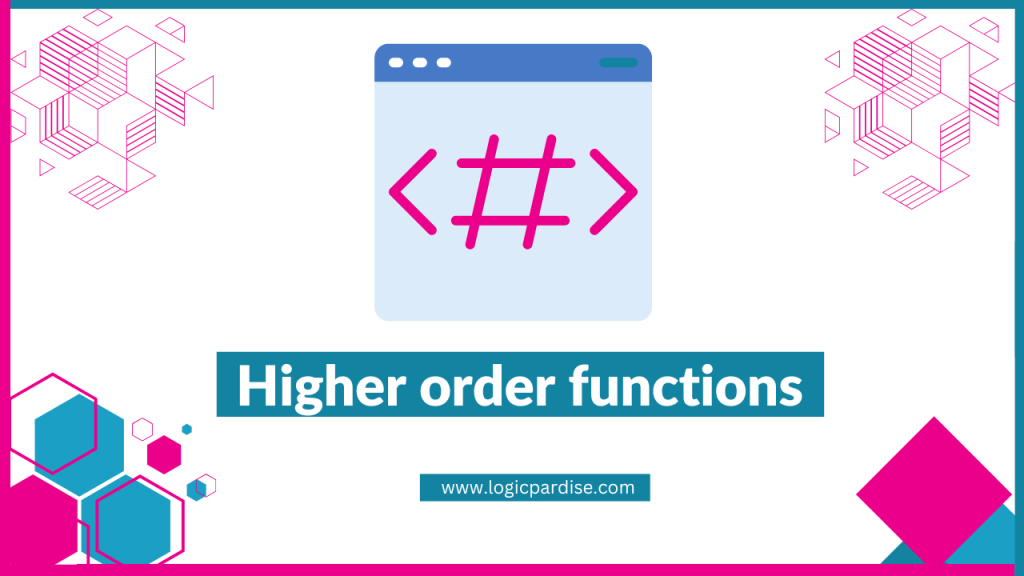
Higher-order functions are a fundamental concept in both JavaScript (JS) and TypeScript (TS). A higher-order function is a function that takes one or more functions as arguments or returns a function as its result. These functions enable more flexible and expressive code by allowing you to treat functions as first-class citizens. Here’s an explanation of higher-order functions with suitable examples in both JS and TS: Higher-Order Functions in JavaScript: In JavaScript, functions are first-class citizens, which means they can be treated like any other data type, such as numbers or strings. Higher-order functions leverage this feature by either accepting functions as arguments or returning functions. Example 1: Functions as Arguments // Higher-order function that takes a function as an argument function operateOnNumbers(x, y, operation) { return operation(x, y); } function add(x, y) { return x + y; } function multiply(x, y) { return x * y; } console.log(operateOnNumbers(5, 3, add)); // Output: 8 console.log(operateOnNumbers(5, 3, multiply)); // Output: 15 In this example, operateOnNumbers is a higher-order function that accepts two numbers and a binary operation function as arguments. Depending on the operation function passed, it performs addition or multiplication. Example 2: Functions as Return Values // Higher-order function that returns a function function greetingGenerator(greeting) { return function (name) { return `${greeting}, ${name}!`; }; } const greetHello = greetingGenerator(“Hello”); const greetHi = greetingGenerator(“Hi”); console.log(greetHello(“Alice”)); // Output: Hello, Alice! console.log(greetHi(“Bob”)); // Output: Hi, Bob! In this example, greetingGenerator is a higher-order function that returns a function. Depending on the greeting passed, it generates a personalized greeting function. Higher-Order Functions in TypeScript: TypeScript builds upon JavaScript’s capabilities and allows you to define types for functions and function parameters, making it easier to work with higher-order functions. Example: Using TypeScript for Type Safety // Higher-order function with type annotations function operateOnNumbers(x: number, y: number, operation: (a: number, b: number) => number): number { return operation(x, y); } function add(x: number, y: number): number { return x + y; } function multiply(x: number, y: number): number { return x * y; } console.log(operateOnNumbers(5, 3, add)); // Output: 8 console.log(operateOnNumbers(5, 3, multiply)); // Output: 15 In TypeScript, you can specify the types of function parameters and return values. This provides type safety and code documentation. Higher-order functions are incredibly useful in functional programming and enable you to write more modular, reusable, and expressive code. They are commonly used with array methods like map, filter, and reduce, as well as in libraries and frameworks that leverage callbacks, promises, and event handling.
Testing a S/W
Testing Levels: Test Case Design: Testing Techniques: Mastering these testing levels, test case design techniques, and testing methodologies is essential for ensuring that software is thoroughly tested, defects are identified, and the software meets user expectations and requirements.
Coding Best Practices:
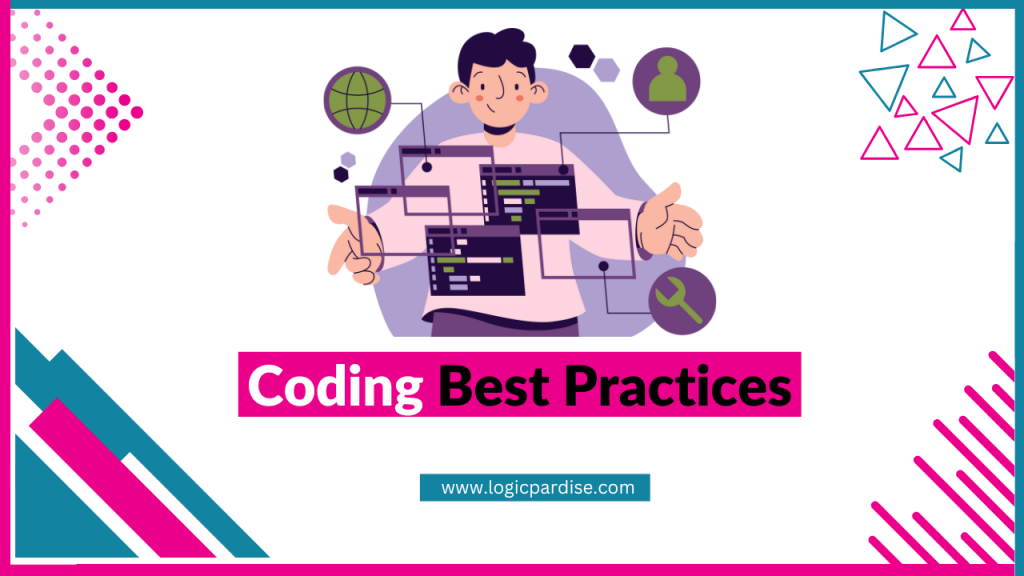
Here’s a comprehensive list of best coding practices along with examples for various programming languages like JavaScript, TypeScript, Python, and more: # Python example def calculate_total(items): total = 0 for item in items: total += item return total // JavaScript example const userAge = 25; function calculateArea(width, height) { return width * height; } // TypeScript example /** * Calculates the area of a rectangle. * @param width The width of the rectangle. * @param height The height of the rectangle. * @returns The area of the rectangle. */ function calculateArea(width: number, height: number): number { return width * height; } # Python example def calculate_tax(income): return income * 0.2 def calculate_net_income(income, deductions): tax = calculate_tax(income) return income – deductions – tax / JavaScript example function calculateTotal(items) { let total = 0; for (const item of items) { total += item; } return total; // TypeScript example class Calculator { static add(a: number, b: number): number { return a + b; } } const sum = Calculator.add(5, 7); // JavaScript example try { const result = someFunction(); } catch (error) { console.error(‘An error occurred:’, error.message); } // TypeScript example function add(a: number, b: number): number { return a + b; } // Test using a testing framework like Jest test(‘adds two numbers’, () => { expect(add(2, 3)).toBe(5); }); # Commit messages in Git git commit -m “Fix issue #123: Refactor user authentication” # Python example def fibonacci(n): if n <= 1: return n return fibonacci(n – 1) + fibonacci(n – 2) // JavaScript example (Express.js with Helmet.js) const express = require(‘express’); const helmet = require(‘helmet’); const app = express(); app.use(helmet()); # Code review feedback “Consider using a more descriptive variable name in line 10.” # Python example def calculate_average(numbers): return sum(numbers) / len(numbers) // TypeScript example class User { firstName: string; lastName: string; } // JavaScript example const TAX_RATE = 0.2; const totalPrice = subtotal + (subtotal * TAX_RATE); # Python example if condition: if another_condition: if third_condition: # … // TypeScript example const user = getUser(); if (user && user.address && user.address.city) { console.log(user.address.city); } // JavaScript example if (userAge >= 18 && userAge <= 65 && !isRetired) { allowAccess(); } # Python example def factorial(n): if n <= 1: return 1 return n * factorial(n – 1) # Update dependencies in package.json (npm) npm update Following these coding practices and examples will lead to writing clean, maintainable, and efficient code across different programming languages, ensuring a higher quality and more productive development process.
.env File and Environments in Node.js
The .env file is a configuration file used to store environment variables for your Node.js application. It’s a text file that contains key-value pairs, where each line represents an environment variable assignment. The purpose of using .env files is to manage application configurations, including sensitive information, without exposing them directly in your codebase. Understanding Environments in Node.js: In Node.js, environments refer to different contexts in which your application runs. The most common environments are “development” (dev) and “production.” Each environment can have its own set of configuration settings, such as database URLs, API keys, and other variables that might differ based on the context. Setting Up .env Files: PORT=3000API_KEY=your_api_key_hereDB_URL=mongodb://localhost/mydb Using .env Files in Node.js: require(‘dotenv’).config(); Access Environment Variables: Once loaded, you can access environment variables using the process.env object. For example: const port = process.env.PORT;const apiKey = process.env.API_KEY;const dbUrl = process.env.DB_URL; Example: Let’s say you’re building a Node.js application with a web server that needs to listen on a specific port and connect to a database. Benefits of Using .env Files: Note: Make sure to include .env in your .gitignore file to avoid exposing sensitive information if you’re using version control like Git.
Environment Variables in Node.js: Managing Application Configurations
Environment variables are a way to store configuration settings and sensitive information outside of your application code. They are particularly useful in Node.js applications to manage various settings such as database connections, API keys, and other configuration details that can vary based on the environment in which the application is running. Understanding Environments: Dev and Production In software development, applications typically have multiple environments: development (dev) and production. Each environment serves a specific purpose: Using Environment Variables Environment variables are set outside of your application code, making them a secure way to manage configuration settings without hardcoding sensitive information. In Node.js, you can access environment variables using the process.env object. This object contains properties corresponding to environment variables. For example, to access an environment variable named API_KEY, you would use process.env.API_KEY. Example: API Key Configuration Let’s say you have an application that interacts with an external API and requires an API key. Instead of hardcoding the API key directly into your code, you can use environment variables. Benefits and Best Practices: Conclusion: Using environment variables in Node.js allows you to manage configuration settings effectively across different environments. By keeping sensitive information separate from your code, you enhance security and ensure that your application behaves consistently across development and production environments.
Debugging option in express
It looks like you’ve provided a detailed excerpt about how Express, a popular Node.js web application framework, uses the debug module for logging purposes. The debug module is used to log information about route matches, middleware functions, application mode, and the flow of the request-response cycle. Unlike console.log, debug logs don’t need to be commented out in production code, as they can be conditionally turned on using the DEBUG environment variable. Here are some key points from the provided text: Remember that the debug module is a powerful tool for development and debugging purposes. It’s helpful for gaining insights into the inner workings of your Express application without cluttering your code with excessive logging statements.
Error Handling in Express
Error handling is a crucial aspect of building robust and reliable web applications in Express. It’s all about managing unexpected issues that might arise during the execution of your code. Let’s break down the concepts in detail and provide easy-to-understand explanations. Catching Synchronous Errors: When you’re dealing with synchronous code (code that executes in a predictable order), Express can automatically catch and process errors that occur. For instance: app.get(‘/’, (req, res) => {throw new Error(‘Something went wrong’); // Express will catch this on its own.}); In this case, if an error is thrown, Express will catch it and handle it appropriately. You don’t need to do anything special. Catching Asynchronous Errors: However, when you’re dealing with asynchronous code (code that involves callbacks, promises, or async/await), you need to handle errors a bit differently. Express won’t automatically catch these errors, so you need to make sure they are passed to Express for proper handling. Here’s how: app.get(‘/’, (req, res, next) => { fs.readFile(‘/file-does-not-exist’, (err, data) => { if (err) { next(err); // Pass errors to Express. } else { res.send(data); } }); }); In this example, if the fs.readFile operation encounters an error, you pass that error to Express using the next function. This tells Express to handle the error using its error-handling mechanisms. Using Promises for Error Handling: Starting from Express 5, if a route handler or middleware that returns a Promise rejects or throws an error, Express will automatically pass that error to the next function. For example: app.get(‘/user/:id’, async (req, res, next) => { const user = await getUserById(req.params.id); res.send(user); }); In this example, if getUserById throws an error or rejects the Promise, Express will automatically catch the error and pass it to the next function. Providing a Custom Error Handler: To handle errors globally, you can define a custom error handler middleware after your routes and other middleware. This middleware has four parameters: (err, req, res, next). app.use((err, req, res, next) => { console.error(err.stack); // Log the error res.status(500).send(‘Something went wrong!’); // Send an error response }); This middleware will be triggered whenever an error is passed to next. The key takeaway is that you must ensure any error that occurs within your route handlers or middleware (synchronous or asynchronous) is either caught and passed to next or handled within your route handler. Remember that effective error handling improves the user experience and helps you identify and address issues in your application more efficiently. Organizing Error Handlers: You can define multiple error-handling middleware functions for different purposes. For instance, you might have a general error logger and a specific client error handler: app.use(logErrors); app.use(clientErrorHandler); app.use(errorHandler); These handlers are organized in the same way as regular middleware. For example, logErrors might log errors to the console, clientErrorHandler handles errors for XHR requests, and errorHandler renders an error page. Skipping Route Handlers: If you want to skip a specific route handler based on certain conditions, you can use the next(‘route’) mechanism: app.get(‘/a_route’, (req, res, next) => { if (!req.user.hasPaid) { next(‘route’); // Skip this route handler } else { next(); // Proceed to the next handler } }, (req, res) => { // This handler will be skipped for unpaid users }); In this example, the second handler will be skipped if the condition isn’t met. Remember, error handling ensures that your application gracefully handles unexpected issues and provides a better user experience. Properly handling errors prevents crashes and helps you troubleshoot problems effectively.
Using template engines with Express
A template engine enables you to use static template files in your application. At runtime, the template engine replaces variables in a template file with actual values, and transforms the template into an HTML file sent to the client. This approach makes it easier to design an HTML page. Some popular template engines that work with Express are Pug, Mustache, and EJS. The Express Application Genreator uses Jade as its default, but it also supports several others. After the view engine is set, you don’t have to specify the engine or load the template engine module in your app; Express loads the module internally, as shown below (for the above example). Create a Pug template file named index.pug in the views directory, with the following content: Then create a route to render the index.pug file. If the view engine property is not set, you must specify the extension of the view file. Otherwise, you can omit it. When you make a request to the home page, the index.pug file will be rendered as HTML. Note: The view engine cache does not cache the contents of the template’s output, only the underlying template itself. The view is still re-rendered with every request even when the cache is on.
Overriding the Express API
The Express API consists of various methods and properties on the request and response objects. These are inherited by prototype. There are two extension points for the Express API: Altering the global prototypes will affect all loaded Express apps in the same process. If desired, alterations can be made app-specific by only altering the app-specific prototypes after creating a new app. Methods You can override the signature and behavior of existing methods with your own, by assigning a custom function. Following is an example of overriding the behavior of res.sendStatus. The above implementation completely changes the original signature of res.sendStatus. It now accepts a status code, encoding type, and the message to be sent to the client. The overridden method may now be used this way:
Third-party Middleware
Third-party Middleware: Third-party middleware are external packages that enhance Express’s functionality. They can be easily integrated into your application. Example: Body parsing middleware using the body-parser package const express = require(‘express’);const bodyParser = require(‘body-parser’);const app = express(); // Third-party middleware for parsing JSON and URL-encoded bodiesapp.use(bodyParser.json());app.use(bodyParser.urlencoded({ extended: true })); app.post(‘/data’, (req, res) => {console.log(req.body);res.send(‘Data received’);}); app.listen(3000, () => {console.log(‘Server is running on port 3000’);});